I’ve been digging around trying to figure out how to get the FTPS status of all of my web apps in an Azure Subscription. Surprisingly there’s not a ton of information about this out there, but it did give me a chance to discover a few tips and tricks to add to my tool belt when working with Azure WebApps.
I’m making some initial assumptions.
1. you’re already logged into Azure. (I’m running this from the Azure PowerShell Cmdlet available inside the Azure Portal GUI.
2. You’re already i the subscription you wanna work with. If you’re not, you can use this
$subscriptionName = “The Name of the subscription you wanna switch to”
Select-AzSubscription -SubscriptionName $subscriptionName
First of all, if you’re wanting to Get a list of all your websites in an azure subscription, you’re can use the command Get-AzureRMResource which will query the Azure Resource Manager for you.
$azureSites = Get-AzureRmResource -ResourceType Microsoft.Web/sites
I created a variable called $azuresites so that I can run a foreach loop and use that variable.
foreach ($azureSite in $azureSites){
$appName = $azureSite.name
$rgName = $azureSite.ResourceGroupName
}
This will simply create a loop that get the webapps from resource manager, and create a couple new variable for my specific needs. appName that just pulls the name of the app, and rgName which grabs to resource group the app is located in. You could write out this just to make sure you’ve got the correct info. Something like this below, but within the brackets for your foreach loop.
foreach ($azureSite in $azureSites){
$appName = $azureSite.name
$rgName = $azureSite.ResourceGroupName
Write-Output $appName $rgName
}
This was all good except what I really wanted to know what more information about the specific site, and it looks like that information was not available directly from the resource manager. To pull app specific information we need to use the cmdlt Get-AzWebApp this will pull details information about your app.
You’d run this call like this (note I’m using the two variable above to supply the application name and that resource group.)
Get-WebApp -Name $appName -ReourceGroupName $rgName
again I added this into for foreach loop:
foreach ($azureSite in $azureSites){
$appName = $azureSite.name
$rgName = $azureSite.ResourceGroupName
Get-AZWebApp -Name $appName -ReourceGroupName $rgName
}
Unfortunately this doesn’t give me all the details that I really really want about an app. In my case, specifically how to get the FTPS state of an web application.
Thankfully we can see all the available child properties of the Get-AZWebApp cmdlet. I did this by create a variable called getwebapp to call the Get-AZWebApp.
$getwebapp = Get-AzWebApp -Name $AppName -ResourceGroupName $rgname
Then I ran $getwebapp. (and tab, and this returned a huge list of available properties I could use.)

The Problem was I still didn’t see any properties that had anything to do with FTPS.
I did find one called SiteConfig though, so I gave that one a try by doing
$getwebapp.SiteConfig and boom that returned a lot of child properties of SiteConfig and one was FTPS.
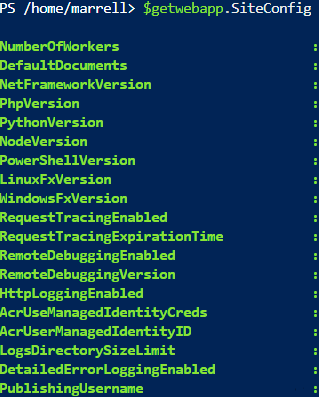

So now I know that I can query this in my loop by adding a new variable called $getwebappSite.Config.FtpsState
So I added that to my loop like this
Draft
Final Script looks like this
$azuresites = Get-AzureRmResource -ResourceType Microsoft.Web/sites
foreach ($azuresite in $azuresites){
$AppName = $azuresite.name
$rgname = $azuresite.ResourceGroupName
#Write-Host “Here is your” $appname $rgname
$getwebapp = Get-AzWebApp -Name $AppName -ResourceGroupName $rgname
$webapp = $getwebapp.Name
$ftpsState = $getwebapp.SiteConfig.FtpsState
Write-output “$($webapp) $($ftpsstate)”
if ($ftpsState -eq “AllAllowed”){
Write-Output “Updating the Web App $($AppName) to use FTPSOnly for FTP”
Set-AzWebApp -Name $AppName -ResourceGroupName $rgname -FtpsState FtpsOnly
$newftpsState = $getwebapp.SiteConfig.FtpsState
Write-Output “Mew FTPS state is $($newftpsState) on $($appName)”
}
}